The Art of Accessing APIs
Everything You Need To Know To Become a Master of APIs
Nov 28, 2020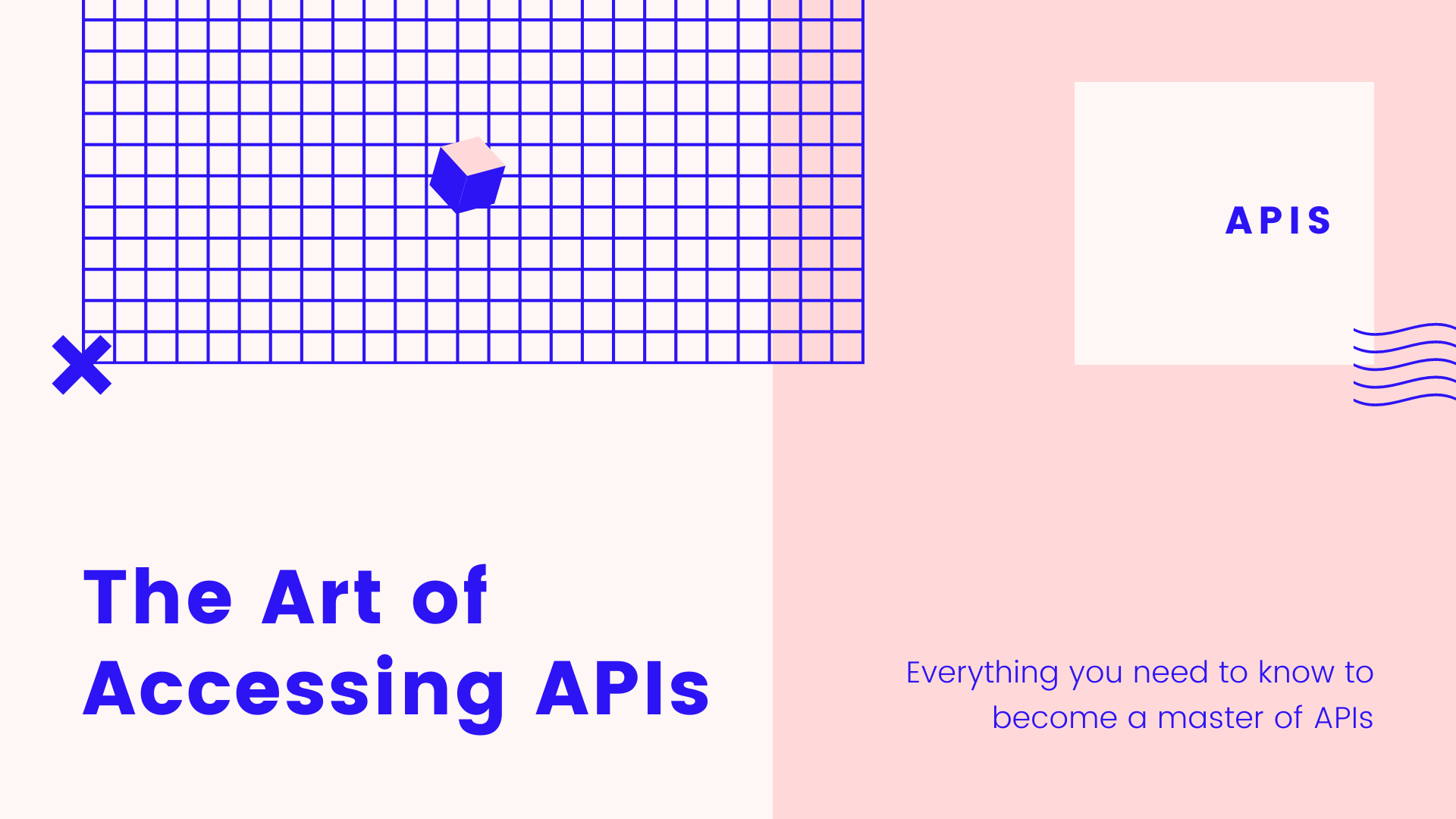
APIs are incredibly powerful and exciting tools that make up a large part of modern technology. Knowing what they are and how to use them is a vital skill every developer should have in their arsenal.
What is an API?
To put it plainly, an API or 'Application Programming Interface' is an intermediary software that allows for communication between two applications. Think of them like a waiter. You sit down at a restaurant and order some food from the menu (you are an application), you convey your order request to the waiter (the API), who then communicates your request for food (data) to the kitchen (the second application). When your food (data) is ready, the waiter brings it straight to your table. That's exactly what an API does - manages requests for data & responses between two applications.
There are a bunch of APIs out there (I listed my favourites here: https://www.elletownsend.co.uk/blog/posts/top-5-apis/, and there is a great list of free public APIs here: https://github.com/public-apis/public-apis). There is no end to the streams of ideas and projects that could stem from utilising these APIs. But if you want to start getting into the wonders of consuming data from APIs, you should read up on how to use them.
That's where this blog post comes in! I want to share my love of APIs with you and cover how to use them so that we can all use APIs and all that fun stuff.
Some Things To Note
There are a few nuances to go through when it comes to approaching APIs for the first time.
When APIs are created, they can be secured using forms of authentication and unique codes that identify who is calling the API. These are put in place so that the owners of the API can keep track of who is using / consuming from the API and prevent malicious use and abuse. Depending on which API you are planning on using, that will determine what you will need to do in order to get access. Most APIs will require you to register for an API key, and use this as an identifier for every call you make to the API. (Note on API keys below)
Secondly, API owners sometimes enforce a limit on the number of requests that can be made to the API in a given time-frame in order to prevent users from overwhelming the service. This is called a 'rate limit', and it varies from API to API.
Documentation is a Dev's Best Friend
You might be thinking, Elle, this is quite a lot of information I need to gather before I access an API, where am I supposed to find it all? I would advise you to get familiar with the API's documentation.
In the documentation of any API, the developers should detail how to get an API key, what the rate limit is (if there is one), and information on the API's endpoints.
When accessing an API, the place your request is sent to and the response is received is called an 'endpoint'. API endpoints come in the form of URLs, and depending on what kind of request you are trying to make, or what data you want back, the API documentation should point you to the endpoint you are looking for.
For example, the endpoint of the Star Wars API that has all the data on films is:
https://swapi.dev/api/films/
and making a request to this endpoint returns information on all Star Wars films.
Know What You're Asking For
The most important thing to consider when writing and debugging your API requests ("Why is this not working?" "I know it's there, why is it undefined??") is what the response you are expecting looks like. The best places to find this out are the API documentation or good old console.log the response and take a look yourself. As you will see in the following code examples, no two APIs are exactly the same, and so the way you access the data from their respective responses will be different too. These little nuances will be the root of lots of your API problems and debugging, but now you know where to look, you should be able to find out how to access that data and use it in your applications.
Now, onto the good stuff…
Making Requests
Search for a Star Wars character by name - (API without an API Key)
Endpoint: https://www.swapi.tech/api/people/
, Query Parameters: name = "r2-d2"
.
Example (JavaScript & Axios)
axios.get('https://swapi.dev/api/people/', { | |
params: { | |
name: 'r2-d2' | |
} | |
}).then(response => { | |
console.log(response.data.results) | |
}); |
Example (Python)
import requests | |
url = "https://swapi.dev/api/people/" | |
response = requests.get( | |
url, | |
params={ | |
"name": "r2-d2" | |
} | |
) | |
data = response.json() | |
print(data['results']) |
Search for a Movie by title - (API with an API Key)
Endpoint: https://api.themoviedb.org/3/search/movie
, Query Parameters: query = "Harry Potter"
.
Example (JavaScript & Axios)
const api_key = 'your api key'; | |
axios.get("https://api.themoviedb.org/3/search/movie", { | |
params: { | |
api_key: api_key, | |
query: "Harry Potter" | |
} | |
}) | |
.then((response) => { | |
console.log(response.data.results); | |
}); | |
Example (Python)
import requests | |
base_url = "https://api.themoviedb.org/3/search/movie" | |
api_key = 'your api key' | |
response = requests.get( | |
url, | |
params={ | |
"api_key": api_key, | |
"query": "Harry Potter" | |
} | |
) | |
data = response.json() | |
print(data['results']) |
A Note on API Keys 🔑
If you're using an API that requires an API key, please keep that key a secret. If you're not planning on uploading your code or hosting your project publicly, you should be fine, but if you are going to upload it to GitHub or host it live then take these steps to keep your API key secret.
In JavaScript (React)
In your project folder, create a file called .env
, a file where we can store environment variables. In this file on the first line, you're going to want to type REACT_APP_API_KEY=
and then paste your API key. No quotes, no ;
Then when you want to use this environment variable in your code, refer to it using process.env.REACT_APP_API_KEY
instead of a string containing your API key. Don't forget to also add your .env
file to your .gitignore
.
In Python
https://medium.com/dataseries/hiding-secret-info-in-python-using-environment-variables-a2bab182eea - This is a great tutorial blog post on using environment variables in Python.
Resources
- Using Axios - https://blog.logrocket.com/how-to-make-http-requests-like-a-pro-with-axios/
- List of lots of APIs - https://github.com/public-apis/public-apis
- The Movie DB API - https://www.themoviedb.org/documentation/api
- Star Wars API - https://www.swapi.tech/
- Using environment variables in React - https://medium.com/better-programming/using-environment-variables-in-reactjs-9ad9c5322408
- Using environment variables in Python - https://medium.com/dataseries/hiding-secret-info-in-python-using-environment-variables-a2bab182eea